2) Click main.xml and add new button to layer
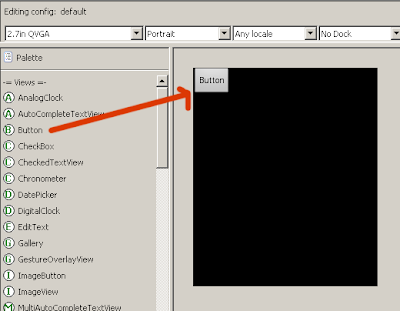
3) If you want to change button variable name right click on that button and select Edit ID and then set variable name
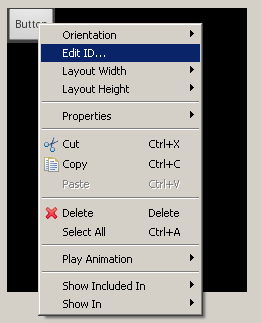
4) If you want to add new text to button right click on that button and select Edit Text. After click that you can see below window.
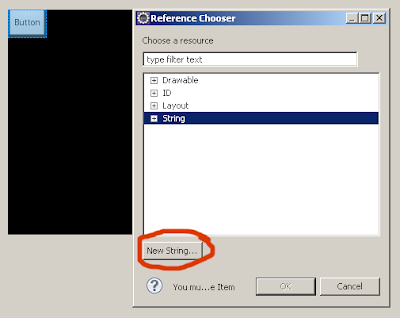
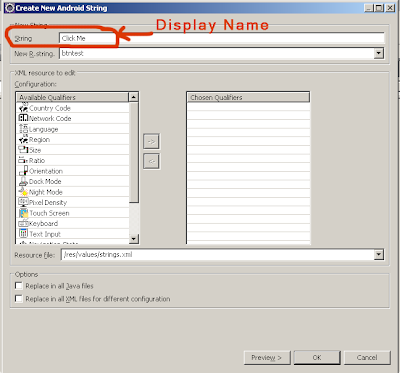
Click Ok and use new sting to your button
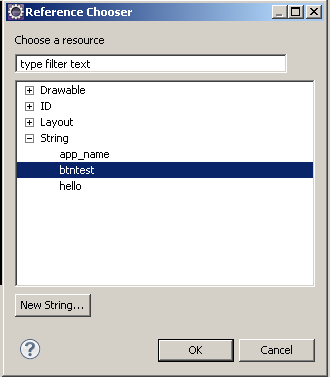
5) Then add below code to your java class as below shown
package lk.cipher.tt;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class TT extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
final Button b=(Button)findViewById(R.id.btnTest);
b.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showToast("Button Clicked");
}
});
}
//show the selected radio channel
private void showToast(String text){
Toast.makeText(TT.this, "" + text, Toast.LENGTH_LONG).show();
}
}
When you click button it display your message "Button Clicked ".
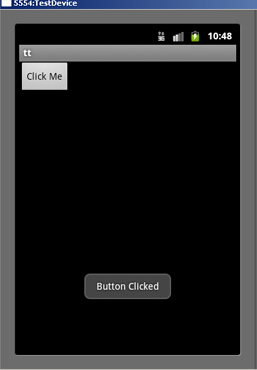
sameeraa4ever